Introduction
Opaque return types, introduced in Swift 5.1, have become a cornerstone feature for modern Swift development, particularly in frameworks like SwiftUI. They enable you to create APIs that expose behavior through protocols while hiding the underlying concrete types, achieving a perfect balance between abstraction and type safety.
This guide covers opaque return types in Swift, explaining their purpose, how they differ from protocols, and how to use them effectively. We’ll also learn about common pitfalls and advanced use cases to help you gain a deeper understanding of this feature.
What Are Opaque Return Types?
An opaque return type allows you to hide the exact type of a return value while still ensuring it conforms to a specific protocol. This is done using the <span class="text-color-code"> some </span>
keyword.
Syntax
func makeShape() -> some Shape {
return Circle(radius: 5.0)
}
In this example, the <span class="text-color-code"> makeShape() </span>
function returns a type conforming to the <span class="text-color-code"> Shape </span>
protocol without revealing the concrete type (<span class="text-color-code"> Circle </span>
) to the caller.
Why Not Just Use Protocols?
At first glance, this might seem no different than returning a protocol directly. However, there are key differences:
Using Protocols
Returning a protocol type allows multiple concrete types to conform and be returned. For example:
protocol Shape {
func draw()
}
func createShape() -> Shape {
if Bool.random() {
return Circle(radius: 5.0)
} else {
return Square(side: 5.0)
}
}
Here, <span class="text-color-code"> createShape() </span>
can return either a <span class="text-color-code"> Circle </span>
or a <span class="text-color-code"> Square </span>
because both conform to <span class="text-color-code"> Shape </span>.
However, this flexibility introduces runtime type erasure:
- The exact type is unknown to the compiler.
- Dynamic dispatch is used to call methods.
- It leads to potential runtime inefficiencies.
Using Opaque Types
With <span class="text-color-code">some</span>, you specify that the function will return one consistent concrete type that conforms to a protocol. For example:
func makeShape() -> some Shape {
return Circle(radius: 5.0)
}
This ensures:
- The compiler knows the exact type (<span class="text-color-code">Circle</span>) at compile time.
- Static dispatch is used, improving performance.
- Type safety is enforced, as you cannot accidentally return multiple concrete types.
Key takeaway: Protocols provide flexibility with multiple types, while opaque types offer type safety and performance by enforcing a single concrete type.
Why Are Opaque Return Types Needed?
Opaque return types address several challenges in API design:
1. Encapsulation
Opaque return types hide implementation details from the caller. This ensures that internal changes, such as switching from one concrete type to another, do not break the API.
2. Static Type Safety
Unlike returning a protocol directly, opaque return types ensure the compiler knows the exact underlying type, enabling better type safety and compile-time error checking.
3. Performance Optimization
Opaque return types avoid type erasure, allowing the compiler to use static dispatch instead of the slower dynamic dispatch associated with protocols.
4. Simplified API Design
Complex or deeply nested types can make APIs cumbersome. By abstracting these details, <span class="text-color-code">some</span> simplifies API usage while preserving flexibility.
Practical Examples
Example 1: Simplifying SwiftUI Views
In SwiftUI, <span class="text-color-code"> some View </span> is extensively used to build user interfaces without exposing the underlying complexity of deeply nested types. Let's break this down for beginners with a more detailed practical example.
Problem Without <span class="text-color-code"> some View </span>
Suppose you want to create a SwiftUI view combining a <span class="text-color-code"> Text </span> and a <span class="text-color-code"> Button </span>. Behind the scenes, SwiftUI composes these views into deeply nested types like <span class="text-color-code"> VStack<TupleView<(Text, Button)>> </span>. Writing this manually would be cumbersome:
struct ContentView: View {
var body: VStack<TupleView<(Text, Button)>> {
VStack {
Text("Hello, World!")
Button("Click Me") {
print("Button tapped!")
}
}
}
}
This is hard to read and maintain. Additionally, the actual type might change if you modify the layout, requiring you to update the return type every time.
Solution With <span class="text-color-code"> some View </span>
Using <span class="text-color-code"> some View </span>, you can simplify the code:
struct ContentView: View {
var body: some View {
VStack {
Text("Hello, World!")
Button("Click Me") {
print("Button tapped!")
}
}
}
}
Now, SwiftUI handles the complexity of the nested types internally, while you work with an abstraction (<span class="text-color-code"> some View </span>) that is simple and consistent.
Example 2: Dynamic Views Based on Conditions
Consider a scenario where you want to display different views based on a condition. Without <span class="text-color-code"> some View </span>, you might run into issues with type mismatches.
Problem With Conditional Views
struct ConditionalView: View {
var showText: Bool
var body: some View {
if showText {
Text("Hello")
} else {
Color.red
}
}
}
This code will throw an error: <span class="text-color-code"> 'some View' requires the body to return a single type. </span> The issue is that <span class="text-color-code"> Text </span> and <span class="text-color-code"> Color </span> are different types.
Fix Using </span> and <span class="text-color-code"> Group </span>
You can use a <span class="text-color-code"> Group </span> to wrap the conditional views, ensuring a consistent return type:
struct ConditionalView: View {
var showText: Bool
var body: some View {
Group {
if showText {
Text("Hello")
} else {
Text("Goodbye")
}
}
}
}
Here, the <span class="text-color-code"> Group </span> ensures that both branches of the <span class="text-color-code"> if </span> statement return the same type (<span class="text-color-code"> Text </span>), which is compatible with <span class="text-color-code"> some View </span>.
Example 3: Reusable View Components
Opaque return types also make it easier to create reusable components in SwiftUI.
Example:
func createButton(title: String, action: @escaping () -> Void) -> some View {
Button(title, action: action)
}
struct ContentView: View {
var body: some View {
VStack {
createButton(title: "Tap Me") {
print("Button tapped!")
}
createButton(title: "Press Me") {
print("Button pressed!")
}
}
}
}
By using <span class="text-color-code"> some View </span>, the <span class="text-color-code"> createButton </span> function hides the exact type of the button while allowing you to reuse it flexibly.
Common Errors with Opaque Return Types
1. Inconsistent Return Types
Opaque return types require a single, consistent concrete type. Returning different types conditionally is not allowed.
Problematic Example:
struct InvalidView: View {
var body: some View {
if Bool.random() {
Text("Hello")
} else {
Color.red
}
}
}
Error: <span class="text-color-code"> 'some View' requires the body to return a single type. </span>
Fix:
Use a wrapper like <span class="text-color-code"> Group </span> to ensure a consistent return type:
struct ValidView: View {
var body: some View {
Group {
if Bool.random() {
Text("Hello")
} else {
Color.red
}
}
}
}
2. Mixing Protocols and Opaque Types
Returning a protocol directly can lead to inefficiencies. Always prefer opaque types when type safety and performance matter.
Advanced Use Cases
1. Using Opaque Types with Generics
Opaque return types can be combined with generics for powerful abstractions.
Example:
func createShape<T: Shape>(_ shape: T) -> some Shape {
return shape
}
let circle = createShape(Circle(radius: 5.0))
circle.draw()
Here, <span class="text-color-code"> createShape </span> can return any type conforming to <span class="text-color-code"> Shape </span> while hiding its concrete type.
2. Type-Specific Views in SwiftUI
You can use opaque types to conditionally return views specific to a type, simplifying complex UI compositions.
Example:
func makeSpecialView<T: View>(for item: T) -> some View {
VStack {
Text("Special View")
item
}
}
struct ContentView: View {
var body: some View {
makeSpecialView(for: Text("Hello World"))
}
}
In this example, the <span class="text-color-code"> makeSpecialView </span> function can compose any <span class="text-color-code"> View </span> with additional UI elements while keeping the API simple.
3. Combining SwiftUI Animations
Use opaque return types to abstract animations while maintaining clean and reusable code.
Example:
func animatedView(show: Bool) -> some View {
VStack {
if show {
Text("Visible")
.transition(.slide)
} else {
EmptyView()
}
}
.animation(.easeInOut, value: show)
}
struct ContentView: View {
@State private var isVisible = false
var body: some View {
VStack {
animatedView(show: isVisible)
Button("Toggle") {
isVisible.toggle()
}
}
}
}
This approach encapsulates animation logic while keeping the code clean and modular.
To sum it up:
Opaque return types (<span class="text-color-code"> some </span>) are a powerful feature that simplifies API design, enhances type safety, and boosts performance. They:
- Hide implementation details while exposing behavior.
- Prevent type erasure and enable static dispatch.
- Simplify working with complex, nested types, especially in frameworks like SwiftUI.
While they may seem complex initially, their advantages make them indispensable in modern Swift development. Mastering opaque return types will significantly enhance your ability to write clean, efficient, and maintainable Swift code.
Send us a quick query
Recommended Posts

OpenAI introduces GPT-4o: The New State of the Art Model
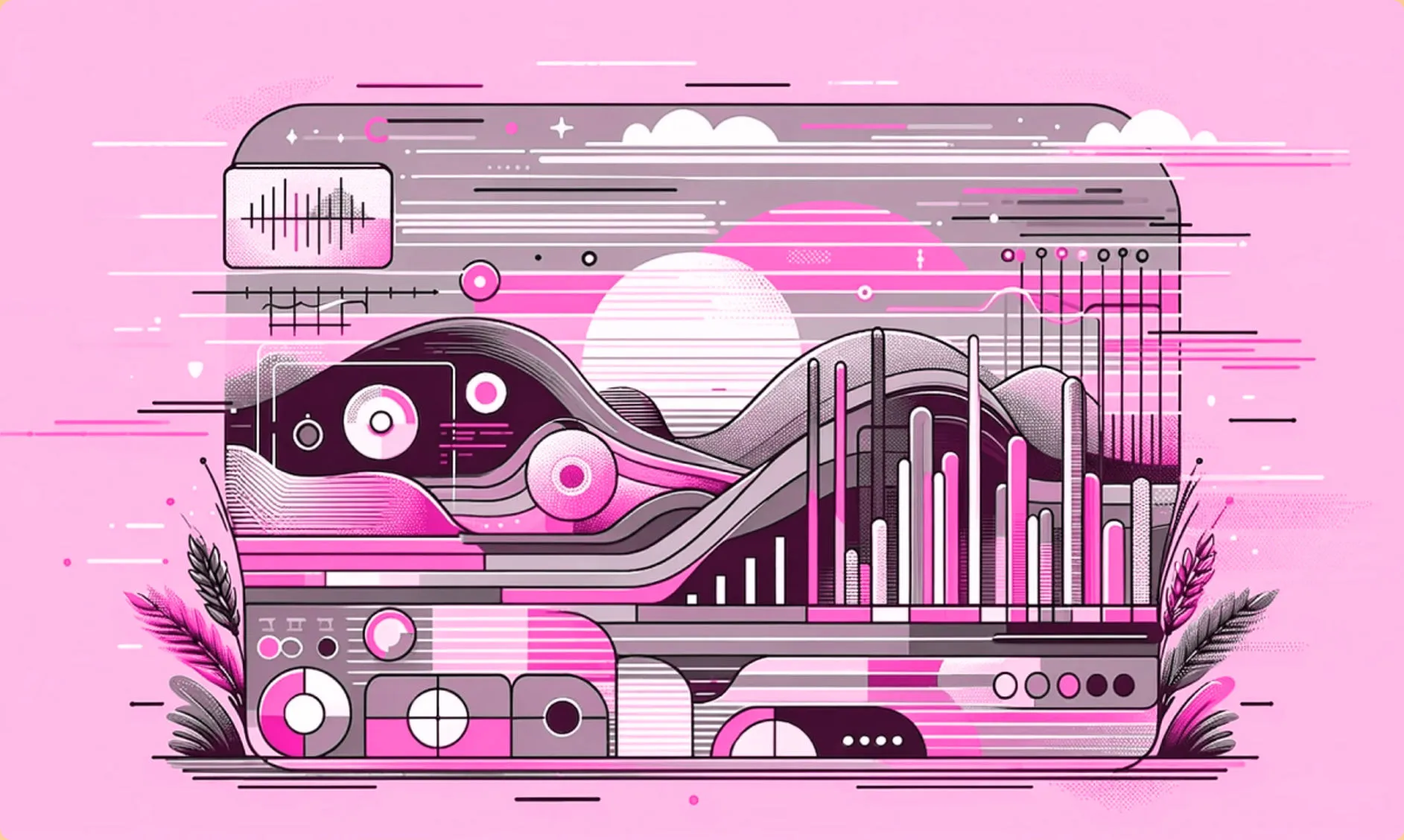
UI Performance Testing Guide In 2023 for Front-end Developers
